Extract Method
Extract Method를 통해 기능마다 함수로 나누어 가독성을 높인다.
- 드래그 후 단축키 Ctrl + R + M *라이더 기준
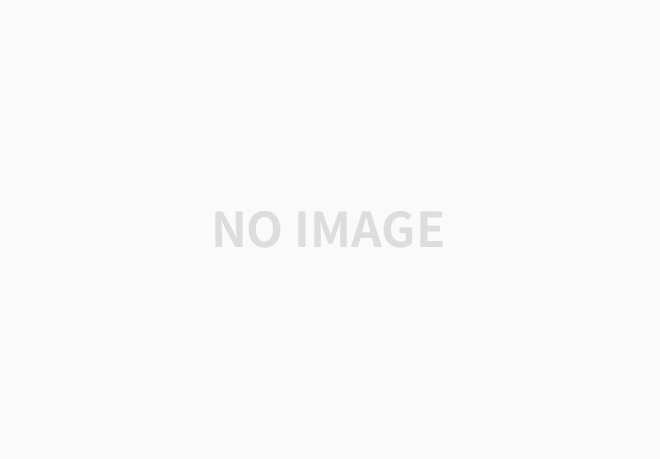
- 이후 이름 짓기 * 나중에 F2 버튼을 통해 다시 지어도 됨 *라이더 기준
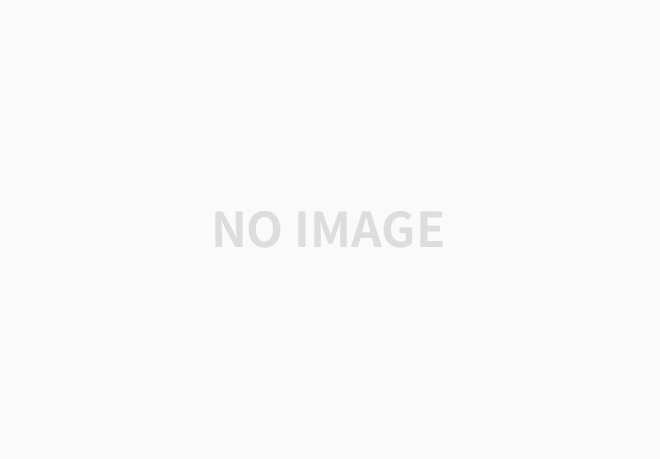
Movement.cs
순서를 맞추면 가독성이 높아진다. Update 안에 ProcessThrust, ProcessRotation -> 이후 순서도 ProcessThrust, ProcessRotation
- 이후에 left right stop 등
using UnityEngine;
using UnityEngine.Serialization;
public class Movement : MonoBehaviour
{
private Rigidbody _rigid;
private AudioSource _audioSource;
[SerializeField] private float mod = 100f;
[SerializeField] private float rotationSpeed = 1f;
[SerializeField] private AudioClip mainEngine;
[SerializeField] private ParticleSystem thrustParticle;
[SerializeField] private ParticleSystem rightThrustParticle;
[SerializeField] private ParticleSystem leftThrustParticle;
void Start()
{
_rigid = GetComponent<Rigidbody>();
_audioSource = GetComponent<AudioSource>();
}
void Update()
{
ProcessThrust();
ProcessRotation();
}
void ProcessThrust()
{
if (Input.GetKey(KeyCode.Space))
{
StartThrusting();
}
else
{
StopThrusting();
}
}
void ProcessRotation()
{
if (Input.GetKey(KeyCode.A))
{
RotateLeft();
}
else if (Input.GetKey(KeyCode.D))
{
RotateRight();
}
else
{
StopRotating();
}
}
void StartThrusting()
{
_rigid.AddRelativeForce(Vector3.up * (mod * Time.deltaTime));
if (!_audioSource.isPlaying)
{
_audioSource.PlayOneShot(mainEngine);
}
if (!thrustParticle.isPlaying)
{
thrustParticle.Play();
}
}
void StopThrusting()
{
thrustParticle.Stop();
_audioSource.Stop();
}
private void RotateLeft()
{
ApplyRotation(rotationSpeed);
if (!rightThrustParticle.isPlaying)
{
rightThrustParticle.Play();
}
}
private void RotateRight()
{
ApplyRotation(-rotationSpeed);
if (!leftThrustParticle.isPlaying)
{
leftThrustParticle.Play();
}
}
private void StopRotating()
{
rightThrustParticle.Stop();
leftThrustParticle.Stop();
}
void ApplyRotation(float rotationThisFrame)
{
// rigid.constraints = (RigidbodyConstraints)((int)RigidbodyConstraints.FreezeRotationX + (int)RigidbodyConstraints.FreezeRotationY);
// rigid.constraints = RigidbodyConstraints.FreezeRotation;
_rigid.freezeRotation = true;
transform.Rotate(Vector3.forward * (rotationThisFrame * Time.deltaTime));
_rigid.freezeRotation = false;
}
}
'유데미 강의 > C#과 Unity로 3D 게임 개발하기 : 부스트 프로젝트' 카테고리의 다른 글
코드로 장애물 움직이기 (0) | 2022.08.23 |
---|---|
치트키와 라이팅 (0) | 2022.08.23 |
파티클 시스템 (0) | 2022.08.23 |
bool 변수로 제어하기, 로켓 꾸미기 (0) | 2022.08.23 |
오디오 클립 (0) | 2022.08.23 |